Understanding Maps
Overview
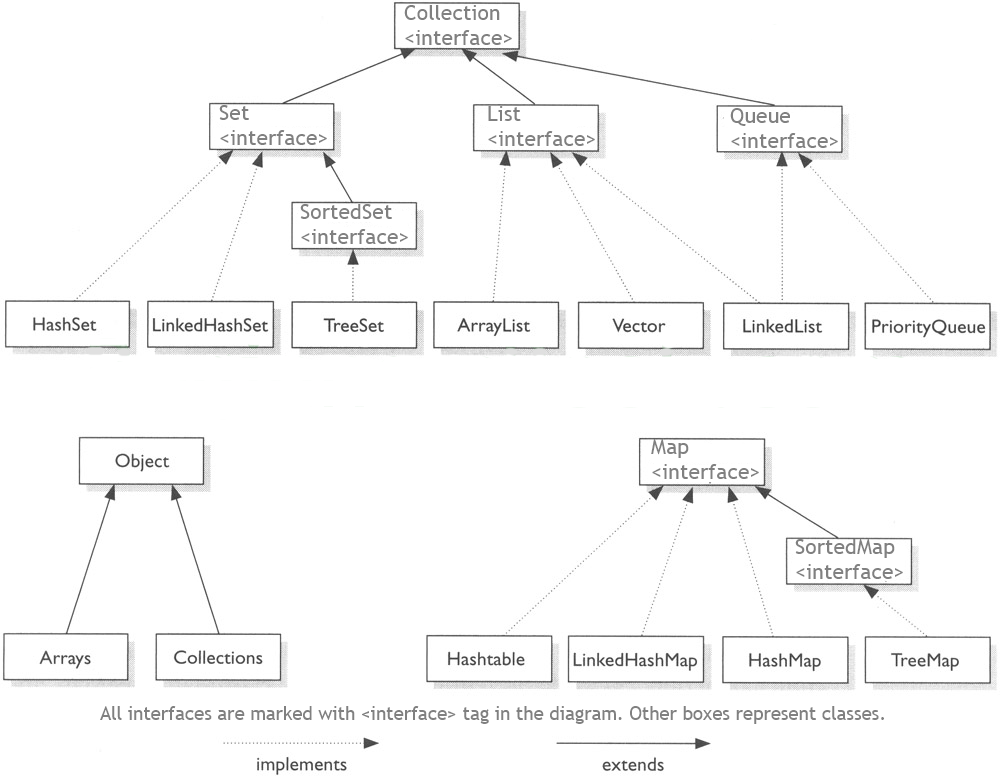
A Map is an object that maps keys to values. A map cannot contain duplicate keys.
Maps: HashMap, TreeMap, LinkedHashMap, WeakHashMap, ConcurrentHashMap, and IdentityHashMap.
They all have the same basic Map interface, but they differ in behaviors including efficiency, the order in which the pairs are held and presented, how long the objects are held by the map, how the map works in multithreaded programs, and how key equality is determined.
The essential methods in a map are put() and get().
HashMap
It makes no guarantees concerning the order of iteration and it’s the fastest among the followings.
TreeMap
It stores its elements in a red-black tree, orders its elements based on their values; it is substantially slower than HashMap.
LinkedHashMap
It orders its elements based on the order in which they were inserted into the set (insertion-order).
WeakHashMap
An entry in a WeakHashMap will automatically be removed when its key is no longer in ordinary use. More precisely, the presence of a mapping for a given key will not prevent the key from being discarded by the garbage collector, that is, made finalizable, finalized, and then reclaimed. When a key has been discarded its entry is effectively removed from the map, so this class behaves somewhat differently from other Map implementations.
This class has performance characteristics similar to those of the HashMap class, and has the same efficiency parameters of initial capacity and load factor.
ConcurrentHashMap
A hash table supporting full concurrency of retrievals and high expected concurrency for updates. This class obeys the same functional specification as Hashtable, and includes versions of methods corresponding to each method of Hashtable. However, even though all operations are thread-safe, retrieval operations do not entail locking, and there is not any support for locking the entire table in a way that prevents all access. This class is fully interoperable with Hashtable in programs that rely on its thread safety but not on its synchronization details.
It divide the big map into N small HashTable (which called segment in the source code) and then put the key into the specific hashtable according to key.hashCode().
IdentityHashMap
This class implements the Map interface with a hash table, using reference-equality in place of object-equality when comparing keys (and values). In other words, in an IdentityHashMap, two keys k1 and k2 are considered equal if and only if (k1==k2). (In normal Map implementations (like HashMap) two keys k1 and k2 are considered equal if and only if (k1==null ? k2==null : k1.equals(k2)).)
This class is not a general-purpose Map implementation! While this class implements the Map interface, it intentionally violates Map’s general contract, which mandates the use of the equals method when comparing objects. This class is designed for use only in the rare cases wherein reference-equality semantics are required.
About Null key and Null value
Both null values and the null key are supported by HashMap, LinkedHashMap, WeakHAshMap, IdentityHashMap.
TreeMap allow null value but not null key while ConcurrentHashMap forbid both null key and null value.
Using Map with Self-define type
If you want to use a self-define type in Map<>, you must override the following two functions.
1 | @Override |